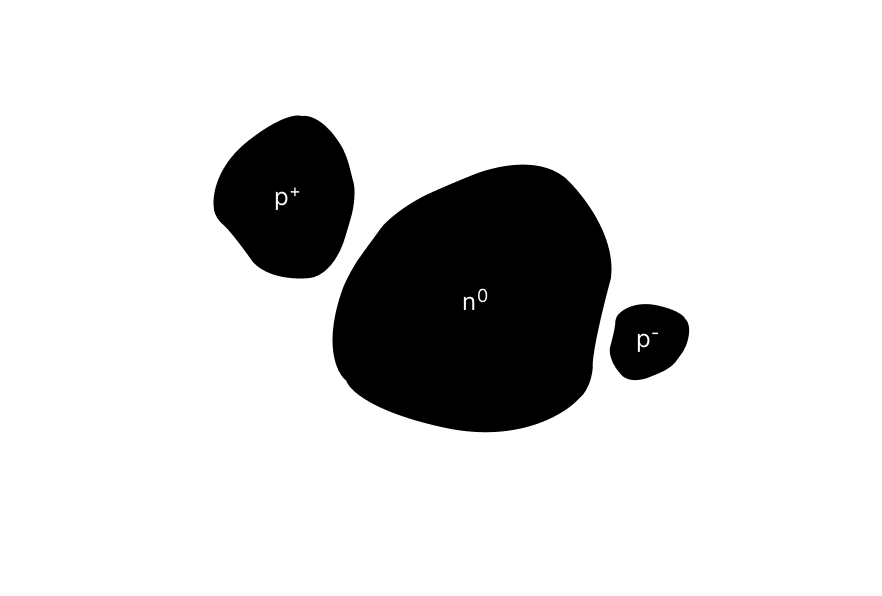
Concept Overview:
A “Learn to Learn” feature would introduce a curiosity-driven, self-improving loop on top of NSAF’s existing capabilities. Currently, NSAF evolves agents for a given objective when instructed; with a Learn-to-Learn extension, the system itself would autonomously seek new knowledge and improvements over time. This can be seen as an outer loop around the current evolutionary process – a loop that decides when and what to learn next, driven by curiosity or an intrinsic reward, rather than being entirely task-driven by external requests.
Proposed Architecture Extension: We can introduce a new component, say a Learning Orchestrator or Meta-Learning Agent, that supervises repeated runs of the Evolution process:
- Lifecycle Hooks: Enhance the Evolution engine to include hooks at key points (e.g. end of each generation, or end of each full evolution run). These hooks would allow a higher-level agent to observe progress and results. For example, after each generation, a hook could compute statistics about the population (diversity, convergence, novel features) and log them to a knowledge store. After an evolution run completes, a hook could trigger analysis of the best agent and how it was achieved.
- Curiosity Module: Implement a module that evaluates the system’s knowledge gaps and formulates new goals. This could be as simple as measuring stagnation – if multiple evolution runs yield similar results, the system might decide to change the task or vary parameters. Or it could be more complex, like generating a new synthetic dataset that challenges the current best agent (for instance, if the agent performs well on one distribution, the orchestrator could create a slightly different task to force the agent to adapt, thereby learning to be more general).
- Daily Scheduled Runs: Utilize a scheduler (in the Node layer or via a persistent Python loop) to trigger learning sessions at regular intervals (e.g., daily). For instance, the MCP server could start a background thread that every 24 hours wakes up and initiates a new evolutionary experiment aimed at improving the agent’s capabilities. The results of each daily run would be fed into the symbolic memory (see below) before the system sleeps until the next cycle. This is analogous to a cron job for self-improvement.
- Symbolic Memory / Knowledge Base: Alongside the neural components, maintain a symbolic memory – a structured record of what has been learned over time. This could be a simple database or file where the system stores outcomes of experiments, discovered rules, or meta-data about agent performance. For example, the system might log entries like: “Architecture X with depth 5 consistently outperforms deeper architectures on task Y” or “Mutation rate above 0.3 caused instability in training”. These pieces of information can be stored in a human-readable format (JSON or even logical predicates) and serve as accumulated knowledge.
- Self-Adaptation: With the above pieces, the orchestrator can now adapt the learning process itself. Using the symbolic memory, the system can adjust its hyperparameters or strategies for the next run – effectively learning how to learn. For example, it might notice that one type of neural activation function often led to better fitness; the next day’s evolution can then bias the initial population to include more of that activation, or update the mutation operators to favor that trait. Alternatively, the system might cycle through different fitness functions or learning tasks to broaden its agents’ skills (a form of curriculum learning decided by the AI itself).
Integration into NSAF MCP Server: To add this feature, we would extend both the Python core and possibly the Node interface:
- Python Side: Create a new class, perhaps
CuriosityLearner
orAutoLearner
, which wraps the Evolution process. It could accept a schedule (number of cycles or a time-based trigger) and manage the symbolic memory. Pseudocode structure: pythonCopyEditclass AutoLearner: def __init__(self, base_config): self.base_config = base_config self.knowledge_db = KnowledgeBase.load(...) # load past knowledge if exists def run_daily_cycle(self): while True: # perhaps check if current time is the scheduled time config = self.modify_config_with_prior_knowledge(self.base_config) evolution = Evolution(config=config) evolution.run_evolution(fitness_function=self.get_curiosity_fitness(), generations=..., population_size=...) best = evolution.factory.get_best_agent() result = best.evaluate(self.validation_data) self.update_knowledge(evolution, best, result) self.save_best_agent(best) sleep(24*3600) # wait a day (or schedule next run)
In this loop,modify_config_with_prior_knowledge
would tweak parameters based on what was learned (for instance, adjustmutation_rate
or choose a different architecture complexity if the knowledge base suggests doing so). Theget_curiosity_fitness
might augment the normal fitness with an intrinsic reward for novelty – e.g., penalize solutions that are too similar to previously found ones, encouraging exploration.update_knowledge
would log the outcome (did the new agent improve? what architectural features did it have? etc.), andsave_best_agent
could maintain a repository of best agents over time (enabling ensemble or recall of past solutions). - Symbolic Memory Implementation: A simple approach could be to use JSON or CSV logs for the knowledge base. Each daily run appends an entry with stats (date, config used, best fitness achieved, architecture of best agent, etc.). Over time, the system can parse this log to find trends. For a more sophisticated approach, one could integrate a Prolog engine or rule-based system to represent knowledge symbolically (e.g., rules like
IF depth>5 THEN performance drops
learned from data). This symbolic reasoning could then be used to explicitly avoid certain configurations or try new ones (for instance, a rule might trigger: “No improvement with current strategy; try increasing input diversity”). - Node/Assistant Integration: The Learn-to-Learn loop can run autonomously once started, but we can also expose controls via MCP. For example, a new MCP tool command like
start_auto_learning
could initiate the AutoLearner background loop, and another likequery_knowledge
could allow the assistant to ask what the system has learned so far (returning a summary of the symbolic memory). Lifecycle hooks would be important to ensure that the assistant is informed of significant events – e.g., after each daily cycle, the system could output a message via MCP indicating “New best agent achieved 5% lower error; architecture features X, Y, Z.” This keeps the human or AI overseer in the loop on the self-improvement progress.
Daily Cycle Example: Suppose the NSAF MCP Server is running continuously on a server with the Learn-to-Learn feature enabled. Each day at midnight, the AutoLearner triggers an evolution run on a reference task (or a set of tasks). The first day, it starts with default settings; it finds, say, a medium complexity network that achieves a certain score. It logs this. By the next day, the symbolic memory has a baseline. The orchestrator now deliberately, out of curiosity, increases the architecture_complexity
to complex and runs again, to see if a deeper network improves performance. If it finds improvement, it logs that deeper was better; if not, it logs that deeper didn’t help. It might also try a completely different synthetic task on day 3 to diversify the agent’s capabilities (ensuring the agent doesn’t overfit to one problem). Over many cycles, the system accumulates knowledge of what architectures and hyperparameters work well under various conditions, effectively tuning its own evolutionary strategy. In doing so it “learns to learn” – it gets better at picking configurations that yield good agents.
Curiosity-Driven Exploration: A core of this feature is intrinsic motivation. We can implement a simple curiosity reward by, for example, favoring agents in the fitness function that exhibit novel behavior or architecture relative to those seen before. Concretely, the fitness_function
could include a term that measures distance from known solutions (one could vectorize an architecture or its performance profile and measure novelty). This means the evolutionary process isn’t just optimizing for an external task (e.g. accuracy on data) but also for surprise or uniqueness. The Knowledge Base aids this by storing fingerprints of past agents. This would gradually expand the variety of solutions the system explores, potentially discovering unconventional architectures that a static fitness alone might miss.
Symbolic Reasoning Integration: Since NSAF is neuro-symbolic, adding a symbolic layer aligns well with its philosophy. For instance, after several runs, the system might infer a symbolic rule like: “IF dataset is small AND layers > 3, THEN overfitting occurs”. The orchestrator could use such a rule to constrain future generations or to decide to apply regularization. This marries the neural search with higher-level reasoning: the symbolic memory acts as the conscience or guide for the otherwise random evolutionary tweaks.
Technical Considerations: Integrating this feature requires careful management of state and process:
- The MCP server would need to remain running persistently (not just per request). We might run the
AutoLearner
in a separate thread or process to not block the main MCP request loop. Alternatively, run the entire MCP server in a persistent mode where it doesn’t exit after a single command but stays alive (the Claude integration config already setsdisabled: false
for the server, implying it can stay residentgithub.com). - Resource management is key – a daily learning loop could be resource-intensive, so the system should either run during idle times or use a reduced workload when running in the background. This could be configured in
Config
(e.g. smaller population for background learning vs. larger if explicitly requested by user). - Checkpointing and persistence become more important: the system should regularly save the state of the AutoLearner (best agents, knowledge base) to avoid losing progress if restarted. The existing
agent.save()
mechanismgithub.com and experiment checkpointing can be leveraged for this. - Feedback Loop with Assistant: With the Learn-to-Learn feature, the AI assistant could even ask the MCP server what it has learned or request it to apply its latest best agent to some user-provided data. This tight coupling means the assistant + NSAF become a more autonomous team: the assistant handles communication and high-level decisions, while NSAF continuously improves its low-level capabilities.
In summary, adding a “Learn to Learn” module would transform the NSAF MCP Server from a one-shot evolutionary tool into a continually self-improving agent framework. It would use lifecycle hooks to monitor itself, schedule regular learning sessions to accumulate improvements, and maintain a symbolic memory of knowledge to drive curiosity and avoid repeating mistakes. For a developer or architect, this extension involves creating new orchestration logic on top of NSAF’s solid foundation: leveraging the modular design to inject higher-level control loops, and using the existing saving, loading, and config systems to support a persistent, evolving knowledge base. The result would be an AI agent that doesn’t just learn once, but keeps learning how to better learn, day after day – pushing the NSAF paradigm toward true continual self-evolution.